The “Foundations of Data Structures and Algorithms Specialization” is a comprehensive program designed to equip learners with a solid understanding of fundamental concepts in computer science, focusing on data structures and algorithms. It lays the groundwork for advanced studies and practical applications in software development, computer science, data analysis, and related fields. Understanding this specialization is vital because data structures and algorithms form the core of problem-solving techniques and efficient software design. By breaking down these concepts into individual courses, learners can systematically grasp the material and apply it to real-world problems.
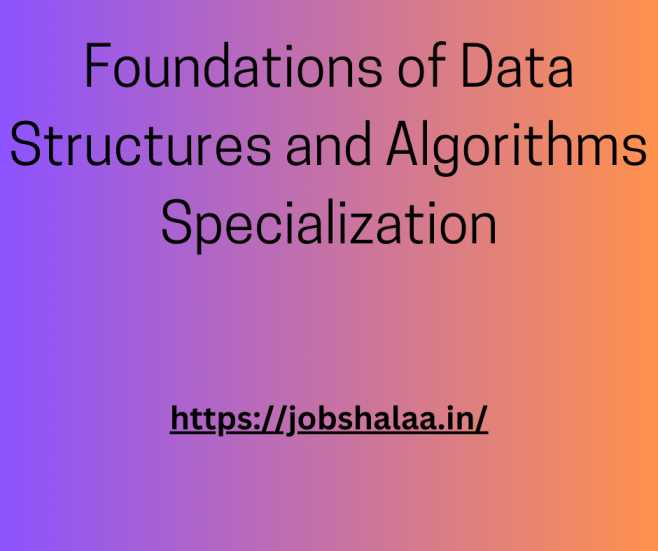
Structure of the Specialization
The specialization typically consists of a series of courses, each focusing on different aspects of data structures and algorithms. It often begins with the basic building blocks, gradually progressing toward more complex topics. The structure can be broken down into the following core areas:
- Introduction to Algorithms and Problem Solving
- Basic Data Structures
- Complex Data Structures
- Algorithmic Techniques
- Advanced Topics and Practical Applications
These courses are designed in a sequence to build upon each other, allowing learners to progressively enhance their understanding and skills. Let’s delve deeper into each area to see how it contributes to the overall learning objectives.
Introduction to Algorithms and Problem Solving
The first part of the specialization typically introduces learners to the fundamentals of algorithms and problem-solving techniques. This section sets the stage by discussing what algorithms are, why they are important, and how they can be used to solve various computational problems.
- Algorithms: Defined as step-by-step instructions for performing a task, algorithms are at the heart of computer programming and problem-solving. This course helps learners understand the basic principles behind writing and analyzing algorithms.
- Problem-Solving Techniques: It also covers different strategies for approaching problems, such as divide and conquer, recursion, and iteration. Understanding these techniques is essential because they form the basis for designing efficient solutions for complex problems.
- Algorithm Analysis: In addition, learners are introduced to the concept of algorithm efficiency, including time complexity (Big O notation) and space complexity. Analyzing algorithms helps in understanding how they will perform under different conditions, which is crucial for optimizing performance.
The knowledge gained in this introductory course provides a foundation that supports the more in-depth study of data structures and advanced algorithms in subsequent courses.
Basic Data Structures
Following the introduction to algorithms, the next set of courses usually dives into basic data structures. Data structures are organized ways of storing and manipulating data to enable efficient operations.
- Arrays and Linked Lists: The first data structures that learners typically encounter are arrays and linked lists. Arrays provide a straightforward way to store elements in contiguous memory locations, which allows for fast access. In contrast, linked lists consist of nodes connected by pointers, offering flexibility in memory management but with slower access times.
- Stacks and Queues: These data structures operate based on specific order principles—Last-In-First-Out (LIFO) for stacks and First-In-First-Out (FIFO) for queues. Learners understand how these structures are used in various applications, such as parsing expressions, managing tasks, or controlling access to shared resources.
- Hash Tables: A hash table allows for efficient searching, insertion, and deletion operations by using a hash function to map keys to specific locations in an array. This data structure is widely used for implementing associative arrays, database indexing, and caching.
Understanding these basic data structures is crucial because they are used to solve a wide variety of problems. Mastery of them ensures that learners can apply the right structure for a given problem, optimizing both time and space efficiency.
Complex Data Structures
Once the basics are covered, the specialization typically advances to more complex data structures, which are suitable for solving intricate problems. These structures are built upon the foundational ones, providing more sophisticated ways of organizing data.
- Trees: Trees are hierarchical data structures consisting of nodes connected by edges. The binary tree, binary search tree (BST), AVL tree, and red-black tree are commonly studied. These trees are important because they enable efficient searching, sorting, and traversal operations. Learners also explore various tree traversal techniques such as in-order, pre-order, and post-order traversal, which are used for different computational tasks.
- Heaps: A heap is a specialized tree-based structure that satisfies the heap property. It is primarily used to implement priority queues, which are useful in scheduling tasks, implementing algorithms like Dijkstra’s shortest path, and for sorting via the heapsort algorithm. There are two types of heaps: min-heaps and max-heaps, each serving different use cases.
- Graphs: Graphs consist of a set of vertices (nodes) connected by edges, which can represent relationships between entities. Graph algorithms such as depth-first search (DFS), breadth-first search (BFS), Dijkstra’s algorithm, and Kruskal’s algorithm are explored in detail. These are applied to solve real-world problems like network routing, social network analysis, and finding shortest paths.
- Tries and Suffix Trees: These are specialized tree structures used in text processing and pattern matching. For example, a trie can be used to implement dictionaries and auto-complete systems, while a suffix tree can be employed for efficient string matching.
Mastery of these complex data structures is essential for tackling advanced algorithms, which require optimized data organization and manipulation.
Algorithmic Techniques
With a solid understanding of data structures, the specialization typically shifts focus to algorithmic techniques. This area is dedicated to learning different methods for designing and implementing algorithms to solve specific types of problems.
- Sorting and Searching Algorithms: Common algorithms such as quicksort, mergesort, bubble sort, binary search, and linear search are discussed in detail. The course emphasizes how to select the appropriate sorting or searching algorithm based on the problem requirements and dataset size.
- Dynamic Programming: Dynamic programming is a technique for solving problems by breaking them down into simpler sub-problems and storing the solutions to avoid redundant calculations. It is used to solve a wide range of optimization problems, including the knapsack problem, longest common subsequence, and coin change problem.
- Greedy Algorithms: A greedy algorithm makes the locally optimal choice at each stage in the hope of finding a global optimum. Greedy techniques are employed in problems such as scheduling, minimal spanning trees, and fractional knapsack.
- Divide and Conquer: This approach involves breaking a problem into smaller sub-problems, solving them independently, and combining their solutions to solve the original problem. Algorithms like mergesort and quicksort utilize divide and conquer principles.
- Backtracking and Branch and Bound: These techniques are used for solving combinatorial and optimization problems by exploring all possible solutions and “backtracking” to eliminate infeasible solutions. Examples include the traveling salesman problem and the N-queens puzzle.
Understanding these algorithmic techniques is fundamental to developing efficient solutions, as different problems require different approaches based on constraints and objectives.
Advanced Topics and Practical Applications
In the final stages of the specialization, learners often explore advanced topics and practical applications. These courses focus on using the knowledge gained from previous courses to solve real-world problems and tackle complex computational tasks.
- Complexity Theory: Complexity theory studies the inherent difficulty of computational problems and classifies them based on the resources required for their solution. Topics like NP-completeness, P vs NP, and computational hardness are covered to give learners a theoretical perspective on algorithm efficiency.
- Parallel Algorithms: With the advent of multi-core processors, parallel computing has become increasingly important. This section explores algorithms that can be divided into independent tasks and executed simultaneously, thereby reducing execution time.
- Application to Real-World Problems: Learners are encouraged to apply data structures and algorithms to solve practical problems. For instance, graph algorithms can be applied to network optimization, while dynamic programming is useful in financial modeling. This helps bridge the gap between theory and practice.
- Coding Challenges and Competitions: Participating in coding challenges or competitions (such as Codeforces, LeetCode, or HackerRank) is encouraged. This enables learners to test their skills, learn from others, and improve their ability to solve problems under time constraints.
The Benefits of Completing the Specialization
Completing the “Foundations of Data Structures and Algorithms Specialization” has several benefits for learners. It provides a structured way to gain a deep understanding of fundamental concepts, which are essential for software development, competitive programming, and technical interviews.
Moreover, it develops problem-solving skills that are not just limited to coding but extend to thinking logically and approaching problems systematically. The knowledge gained from this specialization serves as a prerequisite for more advanced topics, such as machine learning, artificial intelligence, and big data analytics.
Additionally, understanding data structures and algorithms makes learners more proficient in optimizing code, thereby improving software performance. Employers highly value these skills, making this specialization a significant step for anyone aspiring to pursue a career in technology.
Most Important
In conclusion, the “Foundations of Data Structures and Algorithms Specialization” is a valuable learning pathway that provides a thorough grounding in core computer science principles. By covering topics from basic data structures to advanced algorithmic techniques, learners develop the skills necessary to tackle various computational problems effectively. Through a combination of theoretical knowledge and practical applications, this specialization prepares individuals for successful careers in software engineering, data science, and beyond.